In the beginning was the Tao. The Tao gave birth to Space and Time. Therefore Space and Time are the Yin and Yang of programming. — Tao of Programming
Binary Numbers
In decimal system, a number is expressed as a sequence of digits 0 to 9.
For example, Two thousand twenty one ⇔ 2021
In binary number system the set of digits, is called binary digits or bits: {0,1}.
A binary number is expressed as a sequence of bits.
For example, 183 in binary is 10110111.
Converting from decimal to binary
Quotient | Remainder | |
---|---|---|
123 / 2 | 61 | 1 |
61 / 2 | 30 | 1 |
30 / 2 | 15 | 0 |
15 / 2 | 7 | 1 |
7 / 2 | 3 | 1 |
3 / 2 | 1 | 1 |
1 / 2 | 0 | 1 |
Collecting remainders from bottom to top, 123 in binary is 1111011
Converting from binary to decimal
Groups of bits
A group of 8 bits is called a byte e.g. 11010111
- 1 kilobyte (kB) = 1000 bytes
- 1 megabyte (MB) = 106 (million) bytes
- 1 gigabyte (GB) = 109 (billion) bytes
- 1 terabyte (TB) = 1012 bytes (1000 billion)
What is programming?
Programming is the process of creating a set of instructions — a program — to tell a computer how to perform a task.
Programs take input data, perform some computation — numerical or symbolic (text) — and produce output data.
Computers can perform only basic binary operations (such as add or multiply two numbers)
How do we communicate complex instructions to computers? — Use a programming language!
Levels of programming languages
Low-level languages | High-level languages |
---|---|
Closer to machine, difficult for humans | Closer to humans, easier for humans to work with |
Less portable, provide less abstraction over hardware | More portable, more abstraction over hardware |
Examples: Assembly Language | Examples: Java, Python |
How do computers understand high-level languages?
High-level languages are translated into machine code (for CPU).
Programming languages come in two main flavors — compiled languages or interpreted languages.
Compilers and interpreters are software tools responsible to translate source code into machine code.
Compiled languages (e.g. C/C++, Java)
- High-level program (source code) ➞ Compiler ➞ Binary executable (e.g. .exe or .dmg)
- Once compiled, the binary program can be executed without compiler.
Interpreted (e.g. Python, Ruby)
- High-level program (source code) ➞ Executed directly by an Interpreter
- The interpreter is required on the machine where the program is executed.
Data in binary
Computers can understand only binary numbers
How can we encode data in the real world into binary numbers?
Integers in binary
We already saw how to represent positive integers in binary e.g.
For signed integers (to differentiate negative and positive), an extra leftmost bit is used for sign only, e.g.
Real numbers in binary
64-bit Floating point format is used to represent numbers with decimal point, e.g.
Floating point format has a finite precision, but digits of π run forever:
With only 64-bits, we can only have precision up to a fixed digits after decimal point: 3.141592653589793
Text in binary
Letters and punctuations in human languages are encoded in binary using a Character Encoding such as ASCII or UTF-8 (Unicode).
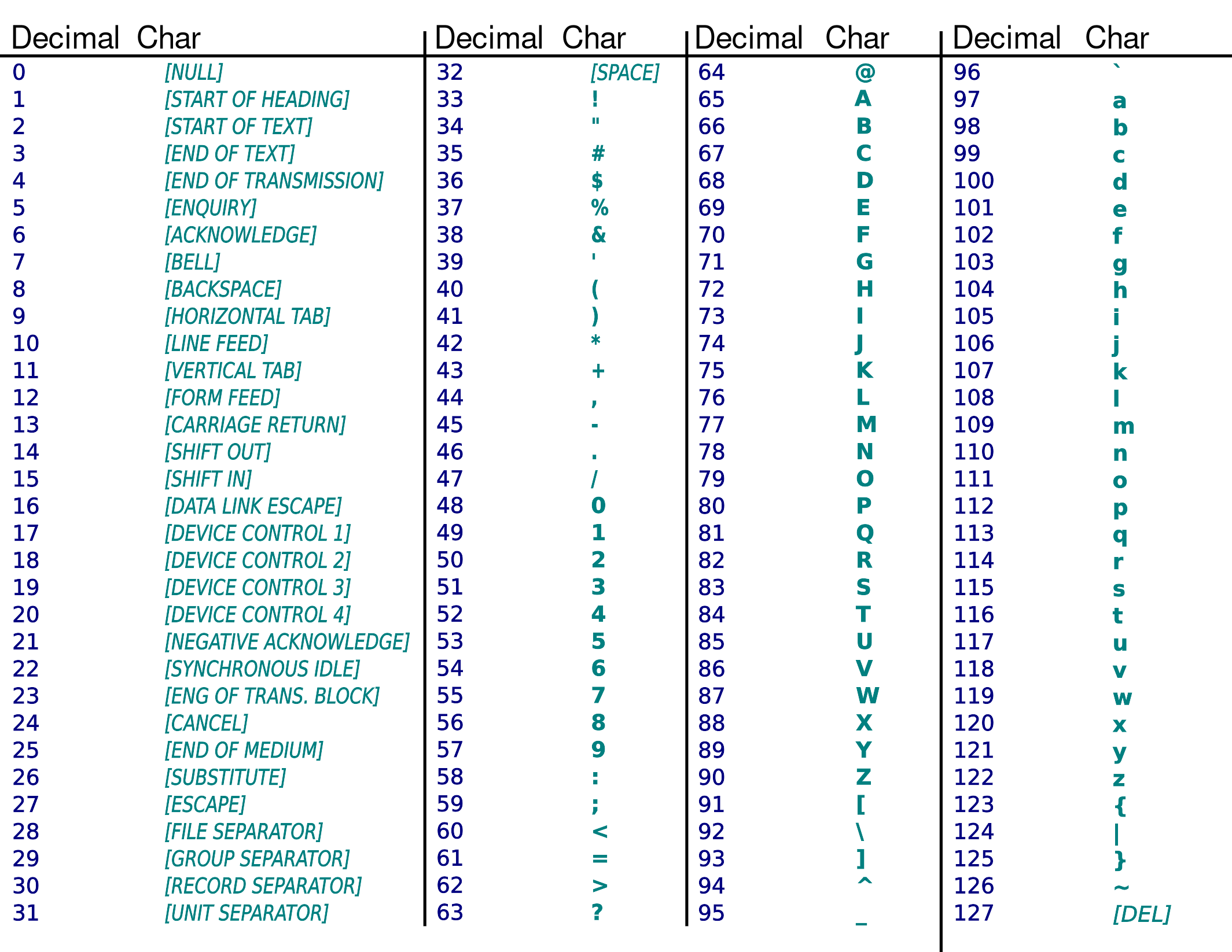
Images, audio & video in binary
Binary data is stored in a file using a specific format.
Programs know what to do (play music, show image, etc) based on the format.
We already know some of these formats:
- Images: jpeg, png
- Audio: mp3, m4a, wma
- Video: mp4, avi, wmv
Thonny Demo — Editor vs Shell
Python interpreter works in two modes:
- An interactive Shell mode (with the prompt >>>)
- Line(s) of code is executed immediately as soon entered and output is visible immediately
- Script mode
- Executes a Python file (.py) as a program.
Thonny allows us to use both modes in one graphical interface.
Comments
Comments are annotations we add to our program and are ignored by the Python interpreter.
In Python, we start a comment using #.
1# Author: Deven2# My first program34# This is a comment on its own line & it will be ignored5print("Hello, world!") # str6print(123) # int7print(1.614) # float
We use comments to:
- Make the code easier to read and understand by explaining how it works.
- Indicate authorship and license.
- Disable some code (prevent it from executing) but still keeping it in the file.
In Thonny, we can use Edit menu -> Toggle comment to comment/uncomment the selected lines.
Objects and Data Types
All data in a Python program is represented by objects.
An object always has a type (or class) associated with it.
We can use type() function to know the type of an object.
>>> type(5)<class 'int'>
>>> type(3.1415)<class 'float'>
>>> type("Hello")<class 'str'>
An object’s type determines the operations that the object supports:
1# objects of int type can be added using +2>>> 10 + 531545# But an object of type str cannot be added to an int using +6>>> "Hello" + 57Traceback (most recent call last):8 File "<pyshell>", line 1, in <module>9TypeError: can only concatenate str (not "int") to str
Summary
We saw the three basic data types in Python:
- int: Integers such as ...,−1,0,1,2,...
- float: Floating-point numbers such as −1.2,3.14, etc.
- str: Text data (a sequence of characters) such as “hello world”, “Python”, etc.
The terms Object and Value are used interchangeably.
So are the terms Class and Type.