Review of last week
All data in a Python program is represented by objects.
An object always has a type (or class) associated with it.
- int: Integers such as ...,−1,0,1,2,...
- float: Floating-point numbers such as −1.2,3.14, etc.
- str: Text data (a sequence of characters) such as “hello world”, “Python”, etc.
In Thonny, we can use Edit menu -> Toggle comment to comment/uncomment the selected lines.
Variables
In Python, a Variable is a name that refers to an object in computer memory.
A variable can be created using Assignment Statement:
variable_name = value
= is known as the assignment operator.
1# create a variable and assign it value 202temperature = 2034# variable temperature refers to 20 which is displayed5print("Today's temperature is", temperature)67# show type of the variable8print("Type of temperature variable is", type(temperature))
Today's temperature is 20 Type of temperature variable is <class 'int'>
Arithmetic with numbers
Calculations with numbers can be done using arithmetic operators.
1# Addition2print(1.5 + 1.5) # 3.034# Subtraction5print(10 - 20) # -10
1# Multiplication2print(42 * 42) # 176434# Division5print(1 / 5) # 0.267# Exponentiation (x to the power of y)8print(2 ** 16) # 65536
1temperature = 202# Unary minus operator3print(-temperature) # -20456# Computing rest mass energy of an electron7rest_mass = 9.109e-31 # Using scientific notation8speed_of_light = 3e8910rest_mass_energy = rest_mass * (speed_of_light ** 2) # E = mc^211print(rest_mass_energy) # 8.198099999999999e-14
Floor division and remainder
1# floor division2print(20 // 3) # 634# remainder5print(20 % 3) # 2
1# Converting seconds to minutes23duration = 3204print(duration, "seconds equal", duration / 60, "minutes.")5# 320 seconds equal 5.333333333333333 minutes.678# Alternative approach:9minutes = duration // 6010seconds = duration % 6011print(duration, "seconds equal", minutes, "minutes and",12 seconds, "seconds.")13# 320 seconds equal 5 minutes and 20 seconds.
Result type of arithmetic operations
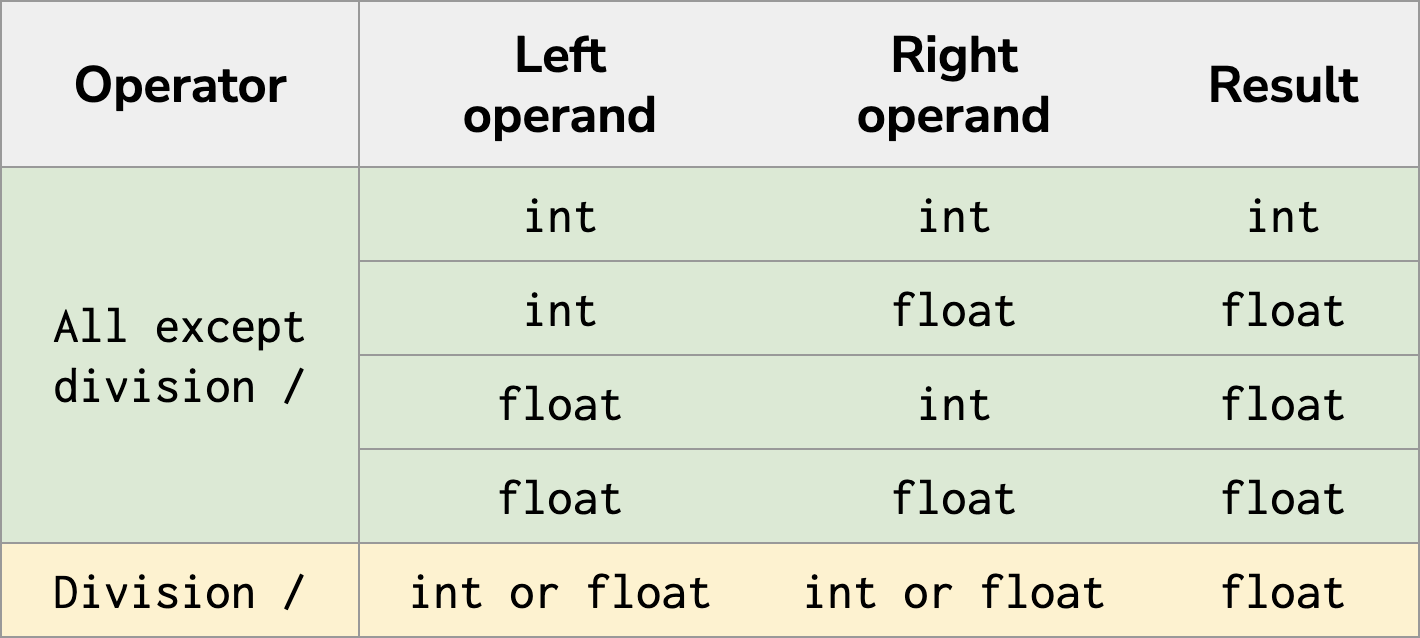
1x = 2 + 12print(x, type(x)) # 3 <class 'int'>34x = 2 + 1.05print(x, type(x)) # 3.0 <class 'float'>67# Classic division always results in float8x = 1 / 29print(x, type(x)) # 0.5 <class 'float'>
Try the above examples with other operators!
Try the problem “Distance between two points” on Ed.
Basic string operations
Strings are sequences of zero or more characters.
In Python, strings are enclosed by either single or double quotes.
1"Hello"2'everyone!'3"I'm Batman." # single quote allowed inside double quotes,4'You can call me "Bruce".' # and vice versa.5'123' # this is a string, not a number!6"" # this is an empty string7" " # this is a string with just one space
1# a multi-line string using triple quotes2lines = """The woods are lovely, dark and deep,3But I have promises to keep,4And miles to go before I sleep,5And miles to go before I sleep.6"""7print(lines)89# We can also use single quotes for multi-line strings10print(11'''I hold it true, whate'er befall;12I feel it when I sorrow most;13'Tis better to have loved and lost14Than never to have loved at all.15''')
String concatenation (joining) using + operator
1message = "Hello" + "everyone"2print(message) # Helloeveryone34name = "Alice"5message = "Hello " + name6print(message) # Hello Alice78string = "1" + "2" + "3"9print(string) # 123 and not the number 61011price = 10012print(price + " USD")13# TypeError: unsupported operand type(s) for +: 'int' and 'str'
String repetition
String can be repeated multiple times using * operator.
1print("Welcome! " * 3) # 'Welcome! Welcome! Welcome! '23print(4 * "ha") # 'hahahaha'
String length
The function len() returns length of its argument string.
1password = "xyz1234"2print("Password length:", len(password))3# Password length: 745print(len(1234))6# TypeError: object of type 'int' has no len()
Order of Expression Evaluation
When we have multiple operators in the same expression, which operator should apply first?
All Python operators have a precedence and associativity:
- Precedence — for two different kinds of operators, which should be applied first?
- Associativity — for two operators with the same precedence, which should be applied first?
Table below show operators from higher precedence to lower.
Operator | Associativity |
---|---|
() (parentheses) | - |
** | Right |
Unary - | - |
*, /, //, % | Left |
Binary +, - | Left |
= (assignment) | Right |
1x = 32y = 53# Multiplication has higher precedence than addition4z = x + 2 * y + 15print(z) # 1467# Need to use parentheses to enforce the order we want8z = (x + 2) * (y + 1)9print(z) # 301011# Same precedence so left to right12z = x * y / 10013print(z) # 0.15
1# Same as 2 ** (3 ** 2) because "**" goes right to left2z = 2 ** 3 ** 23print(z) # 51245# Using parentheses to enforce the order we want6z = (2 ** 3) ** 27print(z) # 6489x = 510x = x + 1 # addition happens first and then assignment11print(x) # 6
More on Variables
Let us write code that implements the following formula to convert fahrenheit to celsius:
c=95(f−32)
1print("10 F in C is", 5 * (10 - 32) / 9)
Variables allow “saving” intermediate results of a computation
We can use variable to store the result so that we can reuse it in the program later.
1fahrenheit = 1023# Store the result of the expression4celsius = 5 * (fahrenheit - 32) / 956print(fahrenheit, "F in C is", celsius)78# Use variable celsius for more calculations9print("Adding 10 degrees today:", celsius + 10)
Variables can be reassigned new values
1# Create variable name "number" and assign a value to it2number = 1233print(number) # displays 12345# Assign new value to existing variable "number"6number = -5078print(number) # displays -50910# add 10 and assign the result value to existing variable "number"11number = number + 101213print(number) # displays -40
New values can be of different type.
However, variables should be changed with caution as it can produce errors or strange results.
1number = 123 # an int value2message = "hello" # a string34# Now variable number refers to the string "hello"5number = message6print(number * 2) # String repetition!7print(number - 10) # minus won't work with string.
hellohello Traceback (most recent call last): print(number - 10) TypeError: unsupported operand type(s) for -: 'str' and 'int'
Example: Swapping values
Sometimes we need to swap (interchange) values of two variables.
A naive attempt (does not work):
1x = 1372y = 4234# Try swapping5x = y6y = x78print(x, y) # 42 42
The following will work:
1x = 1372y = 4234# Correct way to swap5temp = x6x = y7y = temp89print(x, y) # 42 137
Try the problem “Textbox” on Ed.
Rules for variable names
- A variable name can only contain alpha-numeric characters and underscores A-Z, a-z, 0-9, _
- A variable name cannot start with a number
- Variable names are case-sensitive
- (cat, Cat, and CAT are three different variables)
- They cannot be keywords.
- Python has 33 reserved keywords, you can see a list of them by typing help("keywords") in the Python shell.
Python filenames must follow the same rules as above.
Good practice for naming variables
- Name your variable something descriptive of its purpose or content.
- If the variable is one word, all letters should be lowercase. Eg: hour, day.
- If the variable contains more than one word, then they should all be lowercase and each separated by an underscore. This is called snake case.
e.g. is_sunny, cat_name - Good variable names: hour, is_open, number_of_books, course_code
- Bad variable names: asfdstow, nounderscoreever, ur_stupid, CaPiTAlsANyWHErE