Controlling the flow of execution with return statement
As we have seen, return statement allows us to return a value from a function back to the code that calls the function.
But at the same time return statement also ends execution of the function.
When return statement is executed, no further code in the function gets executed.
1def display(message):2 print("*** " + message + " ***")3 return4 print("This will never be displayed")567display("hello")
*** hello ***
return can be very useful when placed inside an if statement if we want to exit from the function under certain conditions.
Using return statement, we can simplify the prime number example:
1# We saw this before2def is_prime(num):3 prime = True45 if num < 2:6 prime = False7 else:8 for i in range(2, num):9 if num % i == 0:10 prime = False11 break1213 return prime
1# Simplified version2def is_prime(num):3 if num < 2:4 return False56 for i in range(2, num):7 if num % i == 0:8 return False910 return True
Important: we must make sure that all branches/cases in the function return the correct values.
In previous example, if we forget the last return statement in the simplified is_prime function, return None will happen implicitly, which would be incorrect.
Be careful — incorrect indentation changes logic (1)
1def remove_spaces(s):2 result = ""3 for i in range(len(s)):4 letter = s[i]5 if letter != " ":6 result = result + letter78 return result
1def remove_spaces(s):2 result = ""3 for i in range(len(s)):4 letter = s[i]5 if letter != " ":6 result = result + letter78 return result
Be careful — incorrect indentation changes logic (2)
1def remove_spaces(s):2 result = ""3 for i in range(len(s)):4 letter = s[i]5 if letter != " ":6 result = result + letter78 return result
1def remove_spaces(s):2 result = ""3 for i in range(len(s)):4 letter = s[i]5 if letter != " ":6 result = result + letter78 return result # what will this do?
ASCII code and special characters
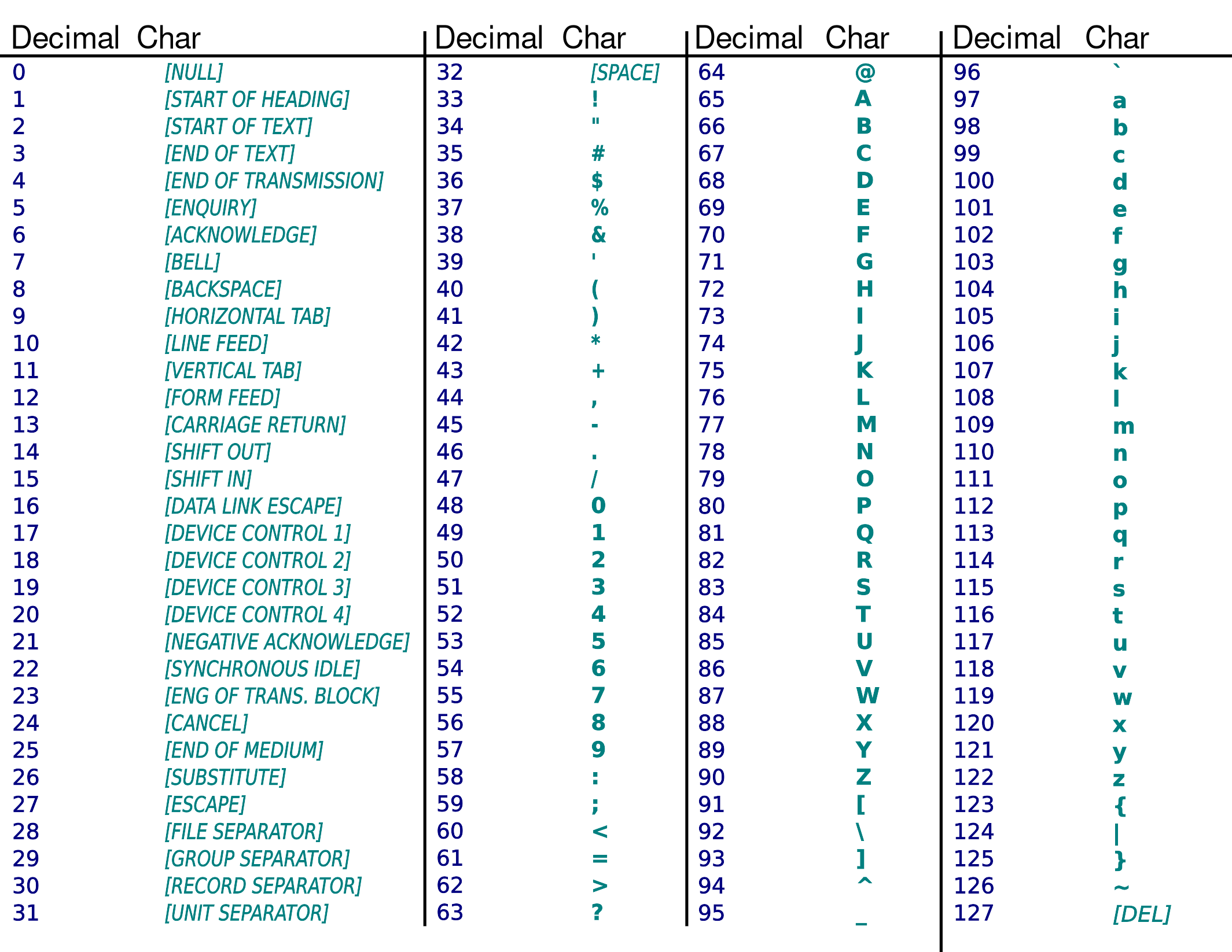
Python has built-in functions to convert ASCII code (decimal) to/from a single character.
1print(ord("a")) # 97, the ASCII code for letter "a"2print(ord("A")) # 653print(ord("$")) # 3645print(ord("hi")) # doesn't work for more than one character6# TypeError: ord() expected a character, but string of length 2 found78print(chr(70)) # F, the character for ASCII code 70910print(chr(103)) # g
Write a program that shifts each letter in a string to the left by 3 steps according to ASCII table.
i.e. A → >, B → ?, C → @, D → A, E → B
, etc.
1word = "Python"23result = ""4for i in range(len(word)):5 code = ord(word[i]) # Get ASCII code for the letter6 code = code - 3 # Shift code by 37 # Get letter for the code and add it to result:8 result = result + chr(code)910print(result) # Mvqelk
Text is stored in a file as a sequence of character codes.
cat dog
Multiple lines in text:
catdog
The newline character, which represents “enter” or “return” key, is also stored when text contains multiple lines.
Escape characters
There are special characters, which we may not directly include in a string, e.g.:
- newline character: This is the character representing “enter” or “return” key.
- tab character: This is the character representing “tab” key.
Such special characters can be used in a string using escape characters.
1# Trying to enter a newline character directly fails:2message = "Hello3world"
message = "Hello ^ SyntaxError: EOL while scanning string literal
To include a newline character in a string we can use the escape character \n in the string:
1message = "hello\nworld"2print(message)
hello world
\n is stored as a single character even though it looks like two.
print(ord("\n")) # 10
Another escape character is \t which represents the tab character.
It is useful as a separator when displaying values:
1# print uses space as separator by default2print("Khalid", 85)3print("Reza", 90)45# Using tab as separator6print("Khalid", 85, sep="\t")7print("Reza", 90, sep="\t")
Khalid 85 Reza 90 Khalid 85 Reza 90
Controlling print() function
In previous example, we used a keyword argument sep= to tell print which separator to use between values.
Unlike the usual arguments, keyword arguments are given in the form name=value; in the example sep is the name of argument and "\t" is the value.
1# separator can be any string2print("Alice", 90, 3.14, sep=",")3# Alice,90,3.14
1print("Alice", 90, 3.14, sep="|")2# Alice|90|3.1434# even longer than one character5print("Alice", 90, 3.14, sep="-----")6# Alice-----90-----3.1478print("Alice", 90, 3.14, sep="") # No separator!9# Alice903.14
By default, print() function displays a newline character \n at end of line.
1print("Good", "morning")2print() # no arguments, just prints "\n"3print(123, 3.14)
Good morning 123 3.14
We can change the end character using another keyword argument to print() function, end=.
1print("A sequence of numbers:")2print(1, end=",")3print(4, end=",")4print(9, end=",")
A sequence of numbers: 1,4,9,
end= is useful in a loop:
1N = 102for i in range(N):3 print(i*i, end=", ") # comma and a space
0, 1, 4, 9, 16, 25, 36, 49, 64, 81,
Change the above example to not print the last comma. E.g.,
0, 1, 4, 9, 16, 25, 36, 49, 64, 81
1N = 102for i in range(N):3 if i == N - 1:4 print(i * i)5 else:6 print(i * i, end=", ")
Multiline strings
Using \n, we can create a single string that contains all of the following lines:
1shopping_list = "Shopping list\n- Milk\n- Eggs\n- Apples\n"2print(shopping_list)
Shopping list - Milk - Eggs - Apples
Python provides a better create multiline strings using triple quotes: ''' or """.
1>>> shopping_list = """Shopping list2- Milk3- Eggs4- Apples5"""6>>> shopping_list7'Shopping list\n- Milk\n- Eggs\n- Apples\n'
Nested Loops
We can have a for/while loop inside other for/while loops.
This is useful when we have two sequences and we need all combinations/pairs of items from the sequences.
Write a program that prints all pairs of numbers that sum to 7 when two six-sided dice are rolled.
1# outer loop for first die d1:2for d1 in range(1, 7):3 # inner loop for second die d24 for d2 in range(1, 7):5 if d1 + d2 == 7:6 print(d1, d2)
1 6 2 5 3 4 4 3 5 2 6 1
Write a program that takes two string—one with consonants and other with vowels—and combines each consonant with every vowel to print a syllable.
1consonants = "bdfghjklmn"2vowels = "aeiou"
ba be bi bo bu da de di do du fa fe fi fo fu ga ge gi go gu ha he hi ho hu ja je ji jo ju ka ke ki ko ku la le li lo lu ma me mi mo mu na ne ni no nu
1consonants = "bdfghjklmn"2vowels = "aeiou"34for i in range(len(consonants)):5 for j in range(len(vowels)):6 syllable = consonants[i] + vowels[j]7 print(syllable, end=" ")8 print() # to start printing from next line
Lists
- A list is like a container that holds a sequence of objects.
- Objects contained in a list are called elements or items.
- Lists are ordered! The order in which the items are stored in the list matters.
- Each item is associated with an index (index 0: first item, index 1: second item, etc.)
Creating a list
A list is created using square brackets, with each item separated by a comma.
1prime_numbers = [2, 3, 5, 7, 11, 13]2print(prime_numbers)3# [2, 3, 5, 7, 11, 13]45print(type(prime_numbers))6# <class 'list'>
A list can contain items of any type. For example we can have a list of strings:
1days = ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"]23# Number of items in the list4print(len(days)) # 756empty_list = []7print(len(empty_list)) # 0
A list can contain any number of items, from zero to as many as the computer’s memory allows.
A list can contains objects of different types.
1# list with mixed types2numbers = [1, 'two', 3.75]
Items of a list don’t need to be unique.
1# list with duplicate values2numbers = [5, "five", 5]
Why use a list?
1# Suppose we want to store grades for multiple students23grades1 = 804grades2 = 1005grades3 = 656# ...7# How many variables?!!89# Use just one variable name "grades"10grades = [80, 100, 65]
Indexing a list
We can access an item inside a list using indexing (square brackets), just as we did for strings.
1days = ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"]2first_day = days[0]3second_day = days[1]4last_day = days[6]5print(first_day, second_day, last_day) # Mon Tue Sun67# No item at index 78print(days[7])9# IndexError: list index out of range
1days = ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"]23# Negative indexing can be used as well4print(days[-1], days[-2]) # Sun Sat56numbers = [1, 'two', 3.75]7print(numbers[0] + numbers[2]) # 4.7589print(numbers[0] + numbers[1])10# TypeError: unsupported operand type(s) for +: 'int' and 'str'
Modifying the content of a list
We can modify the content of a list after it has been created.
We can change a single item using its index and the assignment operator.
1days = ["Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"]2days[0] = "Sun"3print(days)4# ['Sun', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun']56days[7] = "No such day"7# IndexError: list assignment index out of range
for loops are very useful for looping through all the items in a list.
1# compute an average of grades2grades = [85, 78.5, 98, 75, 100]3total = grades[0] + grades[1] + grades[2] + grades[3] + grades[4]4avg = total / 55print(avg) # prints 87.3
For instance, we could use a for loop to compute the average of grades.
1grades = [85, 78.5, 98, 75, 100]23total = 04for i in range(5):5 total += grades[i]67avg = total / 58print(avg) # 87.3
Generalized version:
1grades = [85, 78.5, 98, 75, 100]23total = 04N = len(grades)5for i in range(N):6 total += grades[i]78avg = total / N9print(avg) # 87.3
Try the problem “Dot product” on Ed Lessons.
Try the problem “DNA Cut-site” on Ed Lessons.
Try the problem “Palindrome” on Ed Lessons (from last week).
Try the problem “Smallest and largest divisors” on Ed Lessons (from last week)..